使用 OpenCV for Android 進行圖像特征檢測
android 開發(fā)人員,可能熟悉使用activities, fragments, intents以及最重要的一系列開源依賴庫。但是,注入需要本機功能的依賴關系(如計算機視覺框架)并不像在 gradle 文件中直接添加實現語句那樣簡單!
今天,將專注于使用 OpenCV 庫,將其作為依賴項注入到你的 android 應用程序中。
那么,我們將從這次討論中得到什么?我們將能夠創(chuàng)建一個 android 應用程序并執(zhí)行所需的步驟來集成 OpenCV。
此外,我們將完成一個基于SIFT技術的圖像特征檢測算法。這將是你考慮構建自己的 SDK 的良好起點。
什么是SIFT?
SIFT 代表尺度不變傅立葉變換(Scale Invariant Fourier Transform)。檢測器用于查找圖像上的興趣點。它使我們能夠識別圖像中的局部特征。
SIFT 的優(yōu)勢在于,即使我們大幅縮放圖像,它也能正常工作,因為它將圖像數據轉換為尺度不變坐標。SIFT 使用“關鍵點”來表示圖像中縮放和旋轉不變的局部特征。這是我們將其用于各種應用程序(如圖像匹配、對象檢測、場景檢測等)的基準。
為了識別關鍵點,該算法為你完成:
第 1 步:形成尺度空間——這一步確保特征與尺度無關。
第 2 步:關鍵點定位——這一步有助于識別合適的特征/關鍵點。
第 3 步:方向對齊——這一步確保關鍵點是旋轉后不變的。
第 4 步:關鍵點描述符——這是為每個關鍵點創(chuàng)建描述符的最后一步。
從這里,我們可以使用關鍵點和描述符來進行特征匹配。
現在讓我們設置android項目,
打開Android Studio->New Project->Empty Activity
下載 OpenCV 4.5.1
提取文件夾,然后將 java 文件夾重命名為 OpenCVLibrary451
然后使用 File->New->Import Module 并選擇文件夾
單擊完成。然后,你必須看到該庫已添加到你的項目中。
點擊 File->Project Structure->Dependencies 并選擇 app.
單擊添加依賴項,然后選擇 OpenCVLibrary451
確保選中JDK 11,如果沒有,請轉到 gradle 設置并將目標版本更改為1.8。
我們只需要再添加 JNI 庫,以便調用 SIFT OpenCV 本機函數。將以下內容粘貼到應用程序的構建 gradle 文件中。android下defaultConfig下面
sourceSets{
main {
jniLibs.srcDirs = ['libs']
}
}
然后將幾個文件復制粘貼到你在開始時提取的 opencv 文件夾 [from /OpenCV-android-sdk/sdk/native/libs] 下。轉到項目的 app 文件夾,創(chuàng)建一個名為 libs 的文件夾并粘貼文件。
同樣,在應用程序的主文件夾中創(chuàng)建一個名為 cpp 的文件夾,然后粘貼 /OpenCV-android-sdk/sdk/libcxx_helper 中的文件。你之前提取的那個。
在 android 下 app 的 build gradle 文件中粘貼以下內容
externalNativeBuild {
cmake {
path file('src/main/cpp/CMakeLists.txt')
version '3.18.1'
}
}
同步 grade 文件。如果一切順利,你將看到應用程序的構建。
要測試應用程序,請將 bmp 粘貼到可繪制對象中。我在這里使用了 used test.bmp。
收集 bmp 文件后,將以下內容粘貼到 resources->layout->activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/sample_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello OpenCV Android。。。
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toTopOf="@+id/sample_text"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.641"
app:srcCompat="@drawable/ic_launcher_background" />
</androidx.constraintlayout.widget.ConstraintLayout>
然后,將以下代碼粘貼到 ActivityMain.kt 中
package com.augray.siftandroid
import android.graphics.Bitmap
import android.graphics.BitmapFactory
import android.os.Bundle
import android.view.View
import android.widget.ImageView
import android.widget.TextView
import androidx.appcompat.app.AppCompatActivity
import org.opencv.android.Utils
import org.opencv.core.Mat
import org.opencv.core.MatOfKeyPoint
import org.opencv.features2d.Features2d
import org.opencv.features2d.SIFT
import org.opencv.imgproc.Imgproc
class MainActivity : AppCompatActivity() {
companion object {
// Used to load the 'native-lib' library on application startup.
init {
System.loadLibrary("native-lib")
System.loadLibrary("opencv_java4")
}
}
private var imageView: ImageView? = null
// make bitmap from image resource
private var inputImage: Bitmap? = null
private val sift = SIFT.create()
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
inputImage = BitmapFactory.decodeResource(resources, R.drawable.test)
imageView = findViewById<View>(R.id.imageView) as ImageView
detectAndDrawKeypoints()
}
fun detectAndDrawKeypoints() {
val rgba = Mat()
Utils.bitmapToMat(inputImage, rgba)
val keyPoints = MatOfKeyPoint()
Imgproc.cvtColor(rgba, rgba, Imgproc.COLOR_RGBA2GRAY)
sift.detect(rgba, keyPoints)
Features2d.drawKeypoints(rgba, keyPoints, rgba)
Utils.matToBitmap(rgba, inputImage)
imageView!。畇etImageBitmap(inputImage)
}
}
讓我們看一下上面的一些代碼,以便更好地理解
System.loadLibrary("native-lib")
System.loadLibrary("opencv_java4")
當 cmake 為我們構建所有類并準備就緒時,我們仍然沒有 SIFT 模塊,很遺憾,它移到了新版本 OpenCV 中的其他庫中。
函數 detectAndDrawKeypoints() 獲取位圖并將其轉換為圖像數組(矩陣/多維數組),并使用 SIFT 模塊檢測關鍵點。如果圖像具有良好的對比度、細節(jié)和較少重復的圖案,檢測將產生盡可能多的關鍵點。
構建并運行應用程序
我們剛剛檢測到圖像中的特征。
我們現在可以擴展它來拍攝另一張圖像,獲取它的關鍵點并最終匹配它們以獲得相似性。
原文標題 : 使用 OpenCV for Android 進行圖像特征檢測
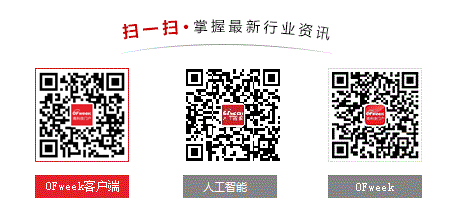
請輸入評論內容...
請輸入評論/評論長度6~500個字
最新活動更多
-
即日-10.29立即報名>> 2024德州儀器嵌入式技術創(chuàng)新發(fā)展研討會
-
10月31日立即下載>> 【限時免費下載】TE暖通空調系統(tǒng)高效可靠的組件解決方案
-
即日-11.13立即報名>>> 【在線會議】多物理場仿真助跑新能源汽車
-
11月14日立即報名>> 2024工程師系列—工業(yè)電子技術在線會議
-
12月19日立即報名>> 【線下會議】OFweek 2024(第九屆)物聯網產業(yè)大會
-
即日-12.26火熱報名中>> OFweek2024中國智造CIO在線峰會
推薦專題
- 高級軟件工程師 廣東省/深圳市
- 自動化高級工程師 廣東省/深圳市
- 光器件研發(fā)工程師 福建省/福州市
- 銷售總監(jiān)(光器件) 北京市/海淀區(qū)
- 激光器高級銷售經理 上海市/虹口區(qū)
- 光器件物理工程師 北京市/海淀區(qū)
- 激光研發(fā)工程師 北京市/昌平區(qū)
- 技術專家 廣東省/江門市
- 封裝工程師 北京市/海淀區(qū)
- 結構工程師 廣東省/深圳市